Introduction
Beehive is one of the fast emerging leaders in providing the companies with the Cloud-based and or an On-premise HRMS software that lists out a number of highly effective features to run HR functions efficiently. The software encompasses key features like Attendance, Leave, Recruitment, Travel, PMS, Payroll, Task Management, Expense, among others, which allows you to automate, streamline, and optimize HR operations for improved employee satisfaction. Based on the specific needs of our clients, we provide them with the software as individual modules and also as an integrated suite for workforce management in both the models – cloud-based and on-premise.
Beehive HCM offers API (Application Programming Interface) for integrating its various modules with any third-party system including web application and mobile applications as well. You can extract employees records and their related various objects data in JSON Format to integrate with your existing business applications like ERP Software, Recruitment Software, Learning and Development Software.
As the APIs are programming language indepent, so you can choose a language of your choice to consume the APIs for integration like C#, Java, PHP, Python, JQuery and Typescript.
API Prerequisites
- Beehive HCM - Subscription: You would require a valid subscription to use API in production environment.
- Developer Account: You need to register for a developer account to use API in development ennvironment.
Developer Account
You need to register for a developer account to use API in development ennvironment.
For Developer account registration, Following are the steps:
- URL: Go to URL https://api.beehivehcm.com/
- Developer Account:Go to Developer Account section and Click on the link.
- Developer Account Registration: Fill the details and click on the Register.
Field | Description |
---|---|
HRMS URL | Here you need to enter your Beehive HRMS URL. Example: (https://hrms.beehivehcm.com), In this case, you need to enter hrms only. |
First Name | Enter your first name |
Last Name | Enter your last name |
Application Name | Enter your application name which will use the APIs |
Application Description | Enter your application description |
Organization Name | Enter your organization name |
Website | Enter your organization website |
Enter your email | |
Mobile Number | Enter your mobile number |
Note: You will receive an email post approval of your account with credentials like username and password.
Authentication
Beehive API's authentication and authorization uses bearer token based authentication which is an HTTP authentication scheme that involves security tokens called bearer tokens. The name “Bearer authentication” can be understood as “give access to the bearer of this token.” The bearer token allowing access to a certain resource or API, generated by the server in response to a login request. The client must send this token in the Authorization header when making requests to Beehive HRMS APIs. The Bearer authentication scheme was originally created as part of OAuth 2.0 in RFC-6750.
Token Generation
You can generate authentication token sending POST request to /oauth/token API with required credentials.
Field | Description |
---|---|
grant_type | password |
username | Provide your username |
password | Provide your password |
Response: You will receive the access_token in response that you need to pass in Authorization header.
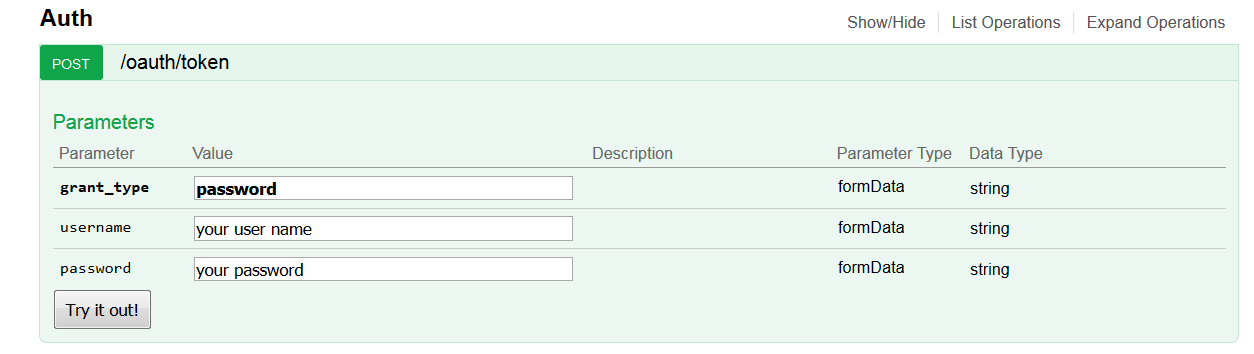
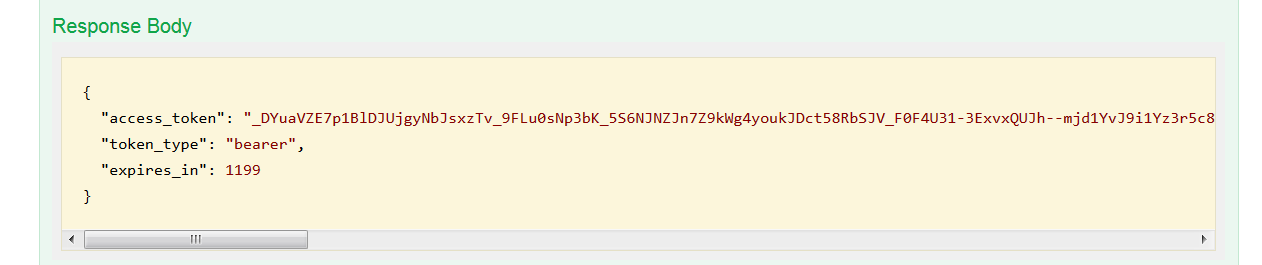
#Create Token class to deserialize the response
using System;
namespace BeehiveHRMS.Client
{
//Token class to deserialize
public class Token
{
public string access_token { get; set; }
public string token_type { get; set; }
public int expires_in { get; set; }
public string error { get; set; }
}
}
#Send request to /oauth/token using HttpClient
using System;
using System.Collections.Generic;
using System.Net.Http;
using System.Web.Script.Serialization;
namespace BeehiveHRMS.Client
{
class Program
{
static void Main(string[] args)
{
string baseAddress = "https://api.beehivehcm.com";
using (var client = new HttpClient())
{
var form = new Dictionary
{
{"grant_type", "password"},
{"username", "your username"},
{"password", "your password"},
};
var tokenResponse = client.PostAsync(baseAddress + "/oauth/token", new FormUrlEncodedContent(form)).Result;
var tokenString = tokenResponse.Content.ReadAsStringAsync().Result;
Console.WriteLine("Response = {0}", tokenString);
var serializer = new JavaScriptSerializer();
var token = serializer.Deserialize(tokenString);
if (string.IsNullOrEmpty(token.error))
{
Console.WriteLine("Token = {0}", token.access_token);
Console.WriteLine("Token Type = {0}", token.token_type);
Console.WriteLine("Expires In = {0}", token.expires_in);
}
else
{
Console.WriteLine("Error = {0}", token.error);
}
}
Console.Read();
}
}
}
#Create Token class to deserialize the response
package com.beehive.beehivehrms;
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
class Token {
@SerializedName("access_token")
@Expose
private String accessToken;
@SerializedName("token_type")
@Expose
private String tokenType;
@SerializedName("expires_in")
@Expose
private Integer expiresIn;
@SerializedName("error")
@Expose
private String error;
public String getAccessToken() {
return accessToken;
}
public void setAccessToken(String accessToken) {
this.accessToken = accessToken;
}
public String getTokenType() {
return tokenType;
}
public void setTokenType(String tokenType) {
this.tokenType = tokenType;
}
public Integer getExpiresIn() {
return expiresIn;
}
public void setExpiresIn(Integer expiresIn) {
this.expiresIn = expiresIn;
}
public String getError() {
return error;
}
public void setError(String error) {
this.error = error;
}
}
#Send request to /oauth/token using HttpURLConnection
package com.beehive.beehivehrms;
import com.google.gson.Gson;
import java.io.IOException;
import java.io.OutputStreamWriter;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class BeehiveHRMSClient {
public static void main(String[] args) throws IOException {
HttpURLConnection connection = (HttpURLConnection) new URL("https://api.beehivehcm.com/oauth/token").openConnection();
connection.setRequestMethod("POST");
String postData = "grant_type=" + URLEncoder.encode("password");
postData += "&username=" + URLEncoder.encode("your username");
postData += "&password=" + URLEncoder.encode("your password");
connection.setDoOutput(true);
OutputStreamWriter wr = new OutputStreamWriter(connection.getOutputStream());
wr.write(postData);
wr.flush();
int responseCode = connection.getResponseCode();
if (responseCode == 200) {
System.out.println("POST was successful.");
BufferedReader br = new BufferedReader(new InputStreamReader(connection.getInputStream()));
StringBuilder sb = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
sb.append(line + "\n");
}
br.close();
System.out.println("Response : " + sb.toString());
//Gson library to deserialize
Gson g = new Gson();
Token token = g.fromJson(sb.toString(), Token.class);
if (token.getError() == null) {
System.out.println("Token : " + token.getAccessToken());
System.out.println("Token Type : " + token.getTokenType());
System.out.println("Expires In : " + token.getExpiresIn());
} else {
System.out.println("Error : " + token.getError());
}
} else if (responseCode == 401) {
System.out.println("Wrong password.");
}
}
}
#Send request to /oauth/token using file_get_contents
<?php
$grant_type = 'password';
$username = 'your username';
$password = 'your password';
$url = 'https://api.beehivehcm.com/oauth/token';
$data = array('grant_type' => $grant_type, 'username' => $username, 'password' => $password);
$options = array(
'http' => array(
'header' => "Content-type: application/x-www-form-urlencoded\r\n",
'method' => 'POST',
'content' => http_build_query($data)
)
);
$context = stream_context_create($options);
$response = file_get_contents($url, false, $context);
if ($response === FALSE) { /* Handle error */
echo "An error has occured.
";
} else {
$json_array = json_decode($response, true);
echo "Access Token: " . $json_array["access_token"];
echo "
Token Type: " . $json_array["token_type"];
echo "
Expires In: " . $json_array["expires_in"];
}
?>